Alright folks, let me tell you about this “money hat” thing I messed around with last week. It’s kinda silly, but hey, sometimes the best learning comes from screwing around, right?
So, I was browsing some random forum, and this idea of a “money hat” popped up. The basic gist is you create a script that watches a cryptocurrency wallet address. When it sees an incoming transaction, BAM, it triggers some action – in my case, I wanted it to display a funny image and play a sound. Why? No good reason, just thought it’d be a fun little project.
First thing I did was pick a cryptocurrency. Went with Dogecoin ’cause it’s cheap and cheerful, and honestly, if I messed something up, it wouldn’t be a huge loss. I fired up my old Raspberry Pi, which I use for random tinkering projects. Figured that’d be a good place to run this thing.
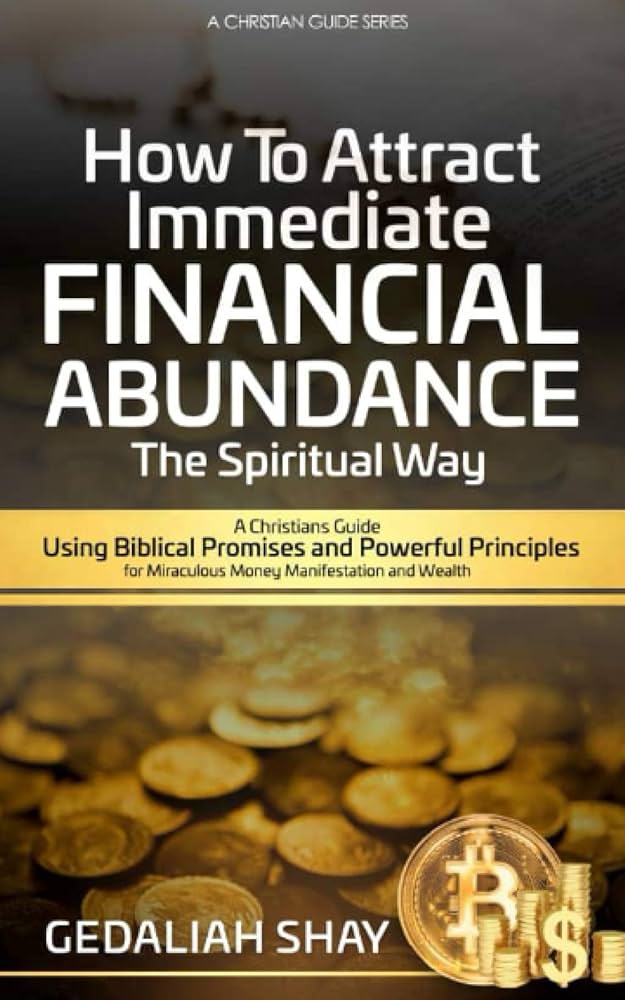
Then came the coding part. Now, I’m no expert programmer, but I can usually hack my way through things. I decided to use Python because I’m somewhat familiar with it, and there are plenty of libraries available. I started by installing the `requests` library to handle the API calls to a Dogecoin block explorer. I chose DogeChain, seemed easy enough to use.
Next, I wrote a simple script that would repeatedly check the balance of my Dogecoin wallet address. I used a `while` loop to keep it running, and a `*()` to avoid hammering the API too much. This part was pretty straightforward.
The tricky part was detecting new transactions. I couldn’t just look at the balance, because the balance might change for other reasons. So, I had to fetch the transaction history. I modified my script to fetch the last few transactions from the DogeChain API. Then, I stored those transaction IDs in a list. Each time the script ran, it would check if any of the new transaction IDs were not in my list of known transactions. If it found a new one, bingo! That meant someone had sent money to my wallet.
Okay, so now I could detect incoming transactions. Time to make the “hat” part happen. I found a ridiculous image of a cat wearing a tiny hat. Perfect. I also grabbed a sound effect – a goofy “ka-ching!” sound. I used the `pygame` library to display the image and play the sound. I had to install `pygame` first, of course: `sudo apt-get install python3-pygame`.
Then I added the code to the script. When a new transaction was detected, it would: 1. Display the cat-hat image. 2. Play the “ka-ching!” sound. 3. Add the new transaction ID to my list of known transactions.
I ran the script… and of course, it didn’t work right away. I had a bunch of syntax errors, and I was using the API wrong. After about an hour of debugging (and a lot of Googling), I finally got it to run without crashing.
To test it, I sent a tiny amount of Dogecoin from my other wallet to my “money hat” wallet. And… it worked! The cat-hat image popped up, the “ka-ching!” sound played. I laughed way harder than I should have.
It’s a dumb project, I know. But it was a fun way to learn a bit more about crypto APIs and Python. And hey, maybe one day I’ll actually make some real money with my money hat. Probably not, but you never know!
Lessons learned:
- APIs are your friend (and sometimes your enemy).
- Debugging is a skill.
- Even silly projects can be educational.